Forwarded headers
The ForwardedHeaders and XForwardedHeaders plugins allow you to handle reverse proxy headers to get information about the original request when a Ktor server is placed behind a reverse proxy. This might be useful for logging purposes.
ForwardedHeaders
handles theForwarded
header (RFC 7239)XForwardedHeaders
handles the followingX-Forwarded-
headers:X-Forwarded-Host
/X-Forwarded-Server
X-Forwarded-For
X-Forwarded-By
X-Forwarded-Proto
/X-Forwarded-Protocol
X-Forwarded-SSL
/Front-End-Https
Add dependencies
To use the ForwardedHeaders
/XForwardedHeaders
plugins, you need to include the ktor-server-forwarded-header
artifact in the build script:
Install plugins
To install the ForwardedHeaders
plugin to the application, pass it to the install
function in the specified module. The code snippets below show how to install ForwardedHeaders
...
... inside the
embeddedServer
function call.... inside the explicitly defined
module
, which is an extension function of theApplication
class.
To install the XForwardedHeaders
plugin to the application, pass it to the install
function in the specified module. The code snippets below show how to install XForwardedHeaders
...
... inside the
embeddedServer
function call.... inside the explicitly defined
module
, which is an extension function of theApplication
class.
After installing ForwardedHeaders
/XForwardedHeaders
, you can get information about the original request using the call.request.origin property.
Get request information
Proxy request information
To get information about the proxy request, use the call.request.local property inside the route handler. The code snippet below shows how to obtain information about the proxy address and the host to which the request was made:
Original request information
To read information about the original request, use the call.request.origin property:
The table below shows the values of different properties exposed by call.request.origin
depending on whether ForwardedHeaders
/XForwardedHeaders
is installed or not.
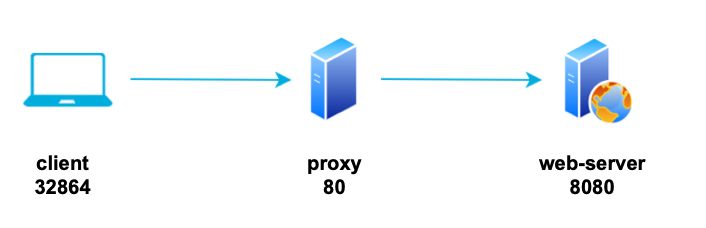
Property | Without ForwardedHeaders | With ForwarderHeaders |
---|---|---|
| web-server | web-server |
| 8080 | 8080 |
| web-server | proxy |
| 8080 | 80 |
| proxy | client |
| 32864 | 32864 |
Configure ForwardedHeaders
You may need to configure ForwardedHeaders
/XForwardedHeaders
if a request goes through multiple proxies. In this case, X-Forwarded-For
contains all the IP addresses of each successive proxy, for example:
By default, XForwardedHeader
assigns the first entry in X-Forwarded-For
to the call.request.origin.remoteHost
property. You can also supply custom logic for selecting an IP address. XForwardedHeadersConfig exposes the following API for this:
useFirstProxy
anduseLastProxy
allow you to take the first or last value from the list of IP addresses, respectively.skipLastProxies
skips the specified number of entries starting from the right and takes the next entry. For example, if theproxiesCount
parameter is equal to3
,origin.remoteHost
will return10.0.0.123
for the header below:X-Forwarded-For: 10.0.0.123, proxy-1, proxy-2, proxy-3skipKnownProxies
removes the specified entries from the list and takes the last entry. For example, if you passlistOf("proxy-1", "proxy-3")
to this function,origin.remoteHost
will returnproxy-2
for the header below:X-Forwarded-For: 10.0.0.123, proxy-1, proxy-2, proxy-3extractEdgeProxy
allows you to provide custom logic for extracting the value from theX-Forward-*
headers.