Docker
In this section we'll see how to deploy a Ktor application to a Docker container which can then be run either locally or on your cloud provider of choice.
Docker is a container system that allows for packaging software in a format that can then be run on any platform that supports Docker, such as Linux, macOS, and Windows. Conceptually Docker is an operating system with layers providing multiple services. While the basics of Docker will be covered, if you're not familiar with it, check out some of the Getting Started documentation.
Getting the application ready
In order to run on Docker, the application needs to have all the required files deployed to the container. As a first step, you need to create a zip file containing the application and its dependencies. Depending on the build system you're using, there are different ways to accomplish this.
The example below will be using Gradle and the application plugin to accomplish this. If using Maven, the same thing can be accomplished using the assembly functionality.
Configure the Gradle file
Prepare Docker image
In the root folder of the project create a file named Dockerfile
with the following contents:
The Dockerfile indicates a few things:
What image is going to be used (JDK 8 in this case).
The exposed port (this does not automatically expose the port which is done when running the container)
How to run the application (
cmd
file)
The other steps merely create a folder, copy the contents from the build output to the folder and change to it in preparation to run the image.
Building and running the Docker image
First step is to create the distribution of the application (in this case using Gradle):
Next step is to build and tag the Docker image:
Finally, start the image:
For more information about running a docker image please consult docker run documentation.
If using IntelliJ IDEA, you can simply click Run
in the Dockerfile to perform these steps.
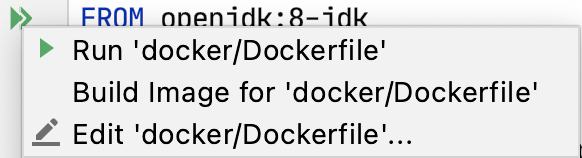